List font layers ↩
This example shows a simple floating window which displays a list of layers in the current font. Whenever the current font changes, the font name and layers list in the UI are updated automatically.
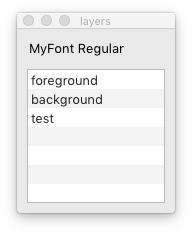
from vanilla import FloatingWindow, TextBox, List
from mojo.subscriber import Subscriber, WindowController, registerRoboFontSubscriber
from mojo.roboFont import CurrentFont
class ListLayersTool(Subscriber, WindowController):
debug = True
def build(self):
self.w = FloatingWindow((200, 200), "layers")
self.w.fontName = TextBox((10, 10, -10, 20), '')
self.w.list = List((10, 40, -10, -10), [])
def started(self):
self.setFont(CurrentFont())
self.w.open()
def destroy(self):
self.w.close()
def fontDocumentDidBecomeCurrent(self, info):
self.setFont(info['font'])
def fontDocumentDidClose(self, info):
self.setFont(CurrentFont())
def setFont(self, font):
if font is None:
self.w.list.set([])
self.w.fontName.set("")
else:
self.w.list.set(font.layerOrder)
fontName = f'{font.info.familyName} {font.info.styleName}'
self.w.fontName.set(fontName)
if __name__ == '__main__':
registerRoboFontSubscriber(ListLayersTool)