Proof spacing ↩
This example shows how to create a simple proof using an installed test font and a generated list of test strings.
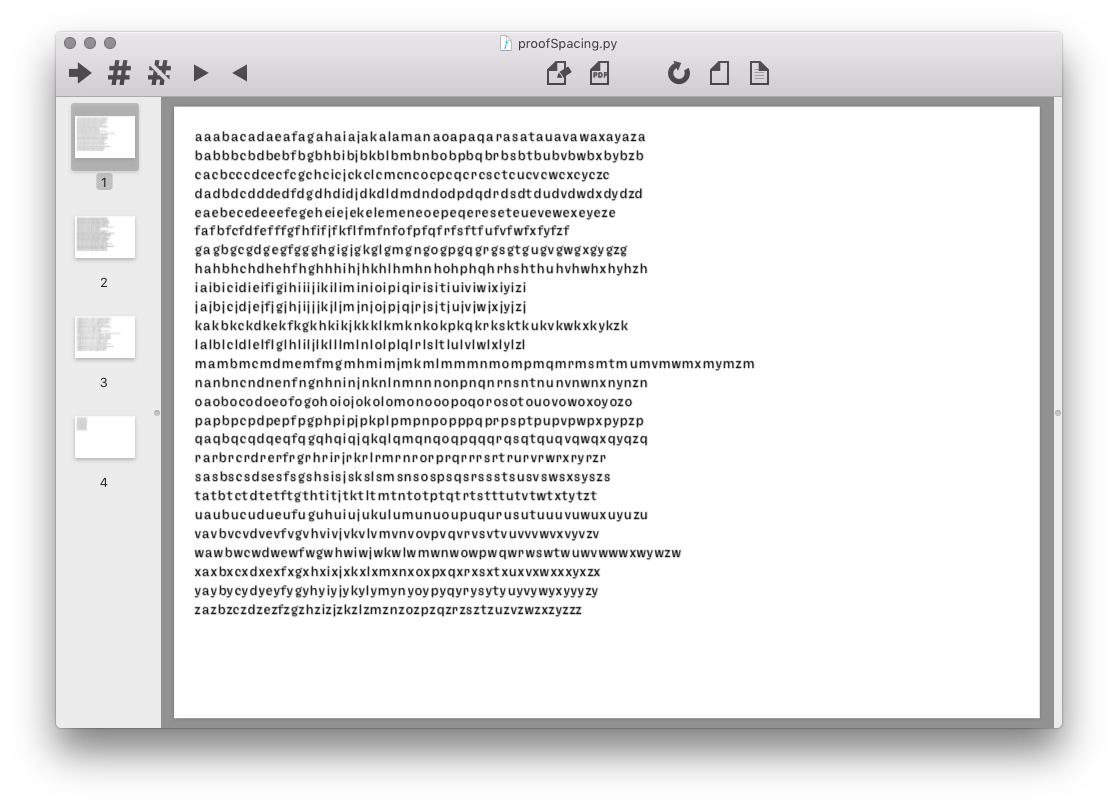
The script works by calling font.testInstall()
to install a test version of the current font locally, so it can be used by the operating system.
The
f.testInstall()
line is commented out because you don’t need to generate and install a font every time you run the script; only when you wish to update to font.
The script then generates test strings for the main groups of characters in a font: lowercase, uppercase, digits, punctuation. These character groups are imported from the string
module, and combined using list comprehensions to produce basic test strings.
from string import ascii_lowercase, ascii_uppercase, digits, punctuation
f = CurrentFont()
fontName = '%s-%s' % (f.info.familyName, f.info.styleName)
# install the font locally
# f.testInstall()
# settings
MARGIN = 20
FONTSIZE = 14
LINEHEIGHT = 1.1 * FONTSIZE
# make some test strings
testStrings = [
''.join(['%s%s%s\n' % (c, c.join(list(ascii_lowercase)), c) for c in ascii_lowercase]),
''.join(['%s%s%s\n' % (c, c.join(list(ascii_uppercase)), c) for c in ascii_uppercase]),
''.join(['%s%s%s\n' % (c, c.join(list(punctuation)), c) for c in ascii_lowercase]),
''.join(['%s%s%s\n' % (c, c.join(list(digits)), c) for c in digits]),
]
# set text with test strings
for txt in testStrings:
# create new page
newPage('A4Landscape')
# set font
if fontName in installedFonts():
font(fontName)
else:
print('font %s not installed' % fontName)
# calculate pos/size
x = y = MARGIN
w = width() - MARGIN * 2
h = height() - MARGIN * 2
# set text sample
fontSize(FONTSIZE)
lineHeight(LINEHEIGHT)
textBox(txt, (x, y, w, h))
This proof can be extended by adding other test strings to the list.