Window Controller ↩
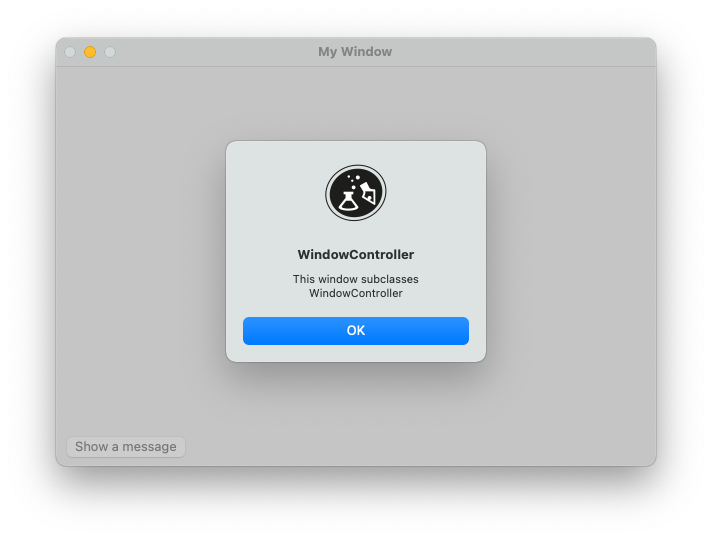
WindowController is basic vanilla window controller that makes common functionalities easily available
WindowController
is a new class shipped with the mojo.subscriber module in RoboFont 4.0. It replaces the defconAppKit
BaseWindow
class. Similarly to the BaseWindow
, WindowController
is a controller of a vanilla
window that adds several common functionalities to a tool that uses a vanilla
Window
or FloatingWindow
. Actions like showing a message, opening a file, or showing a progress bar are immediately available as methods by subclassing WindowController
in your tool. Check the WindowController
reference for a detailed list of methods.
Subscriber
and WindowController
are designed to be compatible in multiple inheritance, so you can benefit from both classes at the same time.
from mojo.subscriber import WindowController, Subscriber
import vanilla
class MyTool(Subscriber, WindowController):
debug = True
def build(self):
self.w = vanilla.Window((600, 400), "My Window")
self.w.button = vanilla.Button(
(10, -30, 120, 20),
"Show a message",
callback=self.buttonCallback)
self.w.open()
def buttonCallback(self, sender):
self.showMessage(messageText="WindowController",
informativeText="This window subclasses WindowController")
if __name__ == '__main__':
MyTool()
By default, you should store your vanilla window into a self.w
attribute. If you want to use another name, just overwrite the getWindow(self)
method and return your vanilla window object.
from mojo.subscriber import WindowController, Subscriber
import vanilla
class MyTool(Subscriber, WindowController):
debug = True
def build(self):
self.window = vanilla.Window((600, 400), "My Window")
self.window.button = vanilla.Button(
(10, -30, 120, 20),
"Show a message",
callback=self.buttonCallback)
self.window.open()
def getWindow(self):
return self.window
def buttonCallback(self, sender):
self.showMessage(messageText="WindowController",
informativeText="This window subclasses WindowController")
if __name__ == '__main__':
MyTool()
WindowController
also allows for a windowWillClose()
callback that is triggered once the window is closed. That’s useful for cleaning merz
sublayers or cleaning any reference to other objects. The following example opens a new font once initiated and closes it along with the window.
from mojo.subscriber import WindowController
from mojo.roboFont import NewFont
import vanilla
class SillyTool(WindowController):
def build(self):
self.w = vanilla.Window((400, 300), "Silly Tool")
self.font = NewFont()
self.w.open()
def windowWillClose(self, sender):
self.font.close()
if __name__ == '__main__':
SillyTool()
If your tool inherits from the Subscriber class, you can override the destroy(self)
method to achieve the same effect
from mojo.subscriber import WindowController, Subscriber
from mojo.roboFont import NewFont
import vanilla
class SillyTool(Subscriber, WindowController):
def build(self):
self.w = vanilla.Window((400, 300), "Silly Tool")
self.font = NewFont()
self.w.open()
def destroy(self):
self.font.close()
if __name__ == '__main__':
SillyTool()
- Intro to Subscriber