Working with large fonts ↩
- Setting the maximum number of glyphs
- Opening Large Fonts warning
- Loading All Glyphs
- Simple font window
This page collects information and tips about working with fonts with a large number of glyphs.
When RoboFont opens a font in the interface, it needs to load data from all glyphs in the font – to render the glyph cells of the Font Overview, and to build the font’s unicode map (used when typing text in the Space Center, for example).
In the UFO format, each glyph is stored as a separate .glif file inside the UFO package; the more glyphs in a font, the more individual files need to be parsed. This is why fonts with a large number of glyphs can have a negative impact on the speed and performance of RoboFont.
One of the ideas under consideration for UFO4 is introducing a new
charactermapping.plist
file to collect the font’s character → glyph name mapping. (The<unicode>
element would be removed from.glif
to prevent duplicate data.) Having a central character map would greatly reduce font loading time for UFO fonts.
To make the opening of large fonts faster, RoboFont has a setting to control how many glyphs are loaded when a font is first opened. The remaining glyphs can be loaded later, if required, by using the Load All Glyphs button.
Setting the maximum number of glyphs
Use the setting Max glyph count to read instantly from disk in the Font Overview Preferences to control the maximum number of glyphs loaded when a font is opened. The default value is 2000 glyphs.
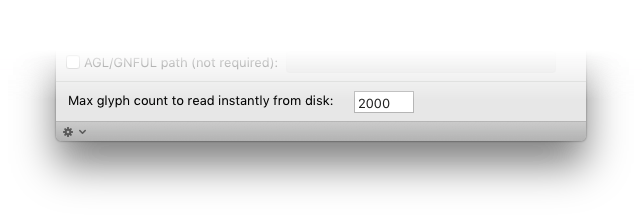
This preference is stored internally as
loadFontGlyphLimit
, and can also be edited with the Preferences Editor.
Opening Large Fonts warning
When opening a font which contains more glyphs than the limit defined in the Max glyph count preference, a pop-up window with a warning will appear:
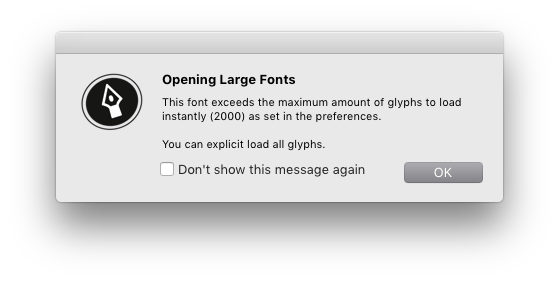
Click on the OK button to close the dialog. Check the box if you don’t want RoboFont to show this message again.
If you have previously selected Don’t show this message again, and wish to undo this choice: go to the Miscellaneous Preferences and click on the button Reset warnings.
Loading All Glyphs
When a font containing more glyphs than the Max glyph count limit is opened, a small button labeled Load All Glyphs will appear at the bottom left of the Font Overview window:
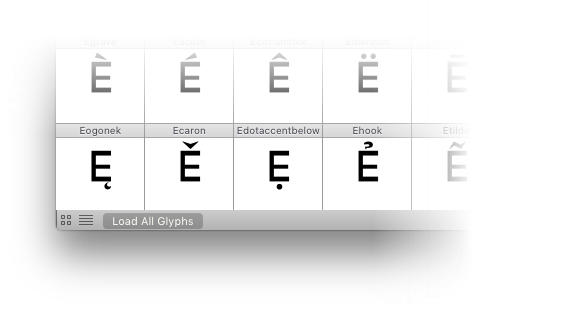
Click the Load All Glyphs button to force RoboFont to load data from all glyphs in the font.
Simple font window
Another approach for dealing with fonts with a large number of glyphs is using a simplified font window. Instead of the usual Font Overview interface with glyph cells, we can use Python to create a simpler (and faster) font window in which the glyphs overview is a simple list of glyph names.
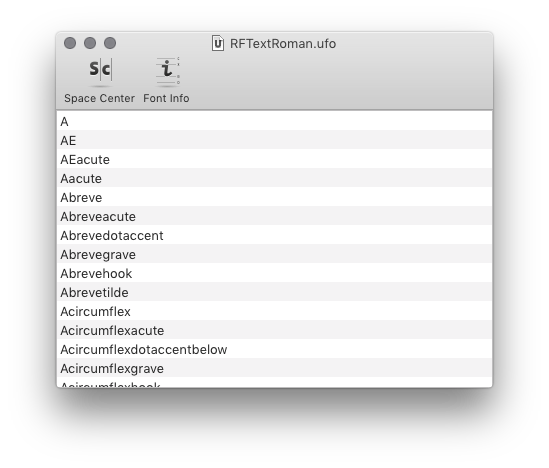
Double-click a glyph name to open a Glyph Editor for that glyph.
from AppKit import NSURL, NSDocumentController
from vanilla import Window, List
from mojo.subscriber import Subscriber, WindowController
from lib.doodleDocument import DoodleDocument
from mojo.UI import OpenGlyphWindow, OpenSpaceCenter, OpenFontInfoSheet
from mojo.roboFont import OpenFont
class SimpleFontWindow(Subscriber, WindowController):
def __init__(self, font):
self._font = font
self._canUpdateChangeCount = True
super().__init__()
def build(self):
self.w = Window((250, 500), "SimpleFontWindow", minSize=(200, 300))
glyphs = sorted(font.keys())
self.w.glyphs = List((0, 0, -0, -0),
glyphs,
doubleClickCallback=self.openGlyph)
toolbarItems = [
dict(itemIdentifier="spaceCenter",
label="Space Center",
imageNamed="toolbarSpaceCenterAlternate",
callback=self.openSpaceCenter
),
dict(itemIdentifier="fontInfo",
label="Font Info",
imageNamed="toolbarFontInfo",
callback=self.openFontInfo
)
]
self.w.addToolbar(toolbarIdentifier="SimpleToolbar", toolbarItems=toolbarItems)
windowController = self.w.getNSWindowController()
windowController.setShouldCloseDocument_(True)
try: # RF >= 3.3
self._font.shallowDocument().addWindowController_(windowController)
except AttributeError:
if not font.path:
return
document = DoodleDocument.alloc().init()
document.setFileURL_(NSURL.fileURLWithPath_(font.path))
dc = NSDocumentController.sharedDocumentController()
dc.addDocument_(document)
self._font.UIdocument().addWindowController_(windowController)
self._font.addObserver(self, "fontChanged", "Font.Changed")
def started(self):
self.w.open()
def openGlyph(self, sender):
sel = sender.getSelection()
if sel:
i = sel[0]
name = sender[i]
self._canUpdateChangeCount = False
OpenGlyphWindow(self._font[name])
self._canUpdateChangeCount = True
def openSpaceCenter(self, sender):
self._canUpdateChangeCount = False
OpenSpaceCenter(self._font)
self._canUpdateChangeCount = True
def openFontInfo(self, sender):
self._canUpdateChangeCount = False
OpenFontInfoSheet(self._font, self.w)
self._canUpdateChangeCount = True
def fontDidChange(self, info):
if self._canUpdateChangeCount:
try: # RF >= 3.3
self._font.shallowDocument().updateChangeCount_(0)
except Exception:
self._font.UIdocument().updateChangeCount_(0)
if __name__ == '__main__':
fonts = OpenFont(showInterface=False)
if not isinstance(fonts, list):
fonts = [fonts]
for font in fonts:
SimpleFontWindow(font)