Glyph Preview ↩
The GlyphPreview object is a simple glyph preview area for use with vanilla windows.
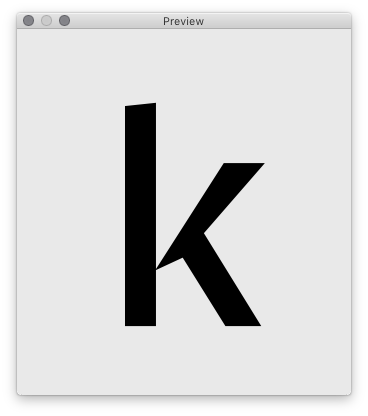
from vanilla import FloatingWindow, List
from mojo.glyphPreview import GlyphPreview
from mojo.roboFont import OpenWindow
class GlyphPreviewExample:
def __init__(self):
# create a window
self.w = FloatingWindow((400, 300), "Preview", minSize=(100, 100))
# add a list for all glyphs in font
self.w.glyphs = List((10, 10, 100, -10), [],
allowsMultipleSelection=False,
selectionCallback=self.showPreviewCallback)
# add a GlyphPreview to the window
self.w.preview = GlyphPreview((100, 0, -0, -0))
# load glyphs from current font to list
self.loadGlyphs(CurrentFont())
# open the window
self.w.open()
def loadGlyphs(self, font):
if not font:
print('to use this tool, please open a font first')
return
# load list of glyphs
self.w.glyphs.set(font.glyphOrder)
def setGlyph(self, glyph):
# set the glyph in the GlyphPreview
self.w.preview.setGlyph(glyph)
def showPreviewCallback(self, sender):
# get selected glyph
selection = self.w.glyphs.getSelection()
if not selection:
return
selectedGlyph = self.w.glyphs.get()[selection[0]]
# set preview in preview
f = CurrentFont()
if f is not None:
self.w.preview.setGlyph(f[selectedGlyph])
# open the window with OpenWindow, so it cannot be opened twice
OpenWindow(GlyphPreviewExample)