Custom label in glyph cells ↩
This example shows how to draw inside the glyph cells of the Font Overview.
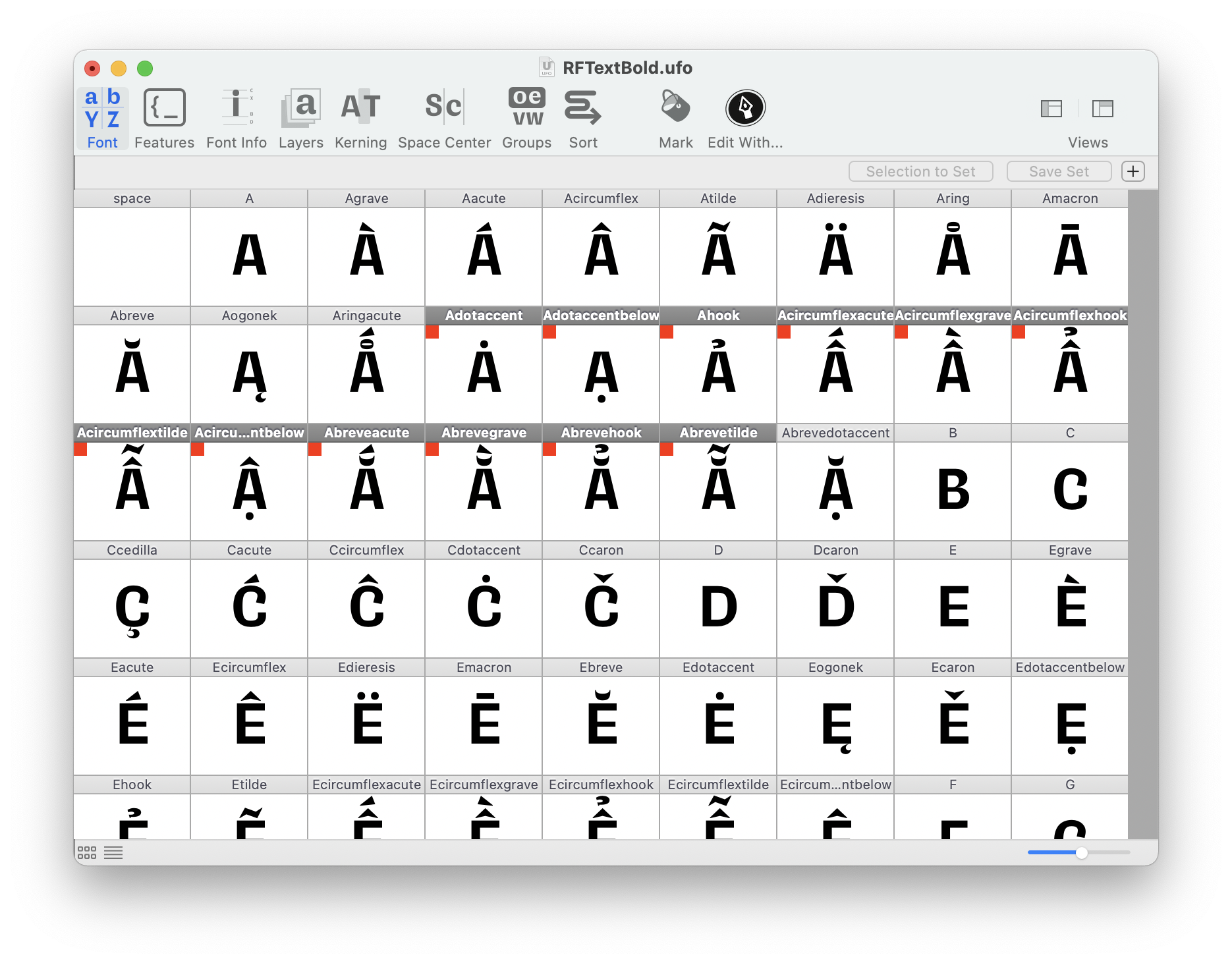
The script opens a dialog containing a button to add or remove a ‘label’ to the selected glyphs in the Font Overview.
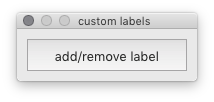
The state of this ‘label’ is stored in a custom key in the glyph lib. When the Font Overview is refreshed, a small rectangle is drawn inside the cells of all glyphs which have this value set to True
.
When the dialog is closed, the observer is removed and the labels are cleared.
from vanilla import FloatingWindow, SquareButton
from mojo.events import addObserver, removeObserver
from mojo.drawingTools import *
class CustomGlyphCellLabel:
key = "com.myDomain.customGlyphCellLabel"
def __init__(self):
self.w = FloatingWindow((180, 52), "custom labels")
self.w.button = SquareButton((10, 10, -10, -10), "add/remove label", callback=self.actionCallback)
self.w.bind("close", self.closeCallback)
self.w.open()
addObserver(self, "drawCell", "glyphCellDrawBackground")
def drawCell(self, info):
glyph = info["glyph"]
value = glyph.lib.get(self.key)
if value:
fill(1, 0, 0)
rect(0, 0, 10, 10)
def actionCallback(self, sender):
font = CurrentFont()
if font is None:
return
for glyphName in font.selectedGlyphNames:
# get current value
value = font[glyphName].lib.get(self.key, False)
# toggle value
font[glyphName].lib[self.key] = not value
font[glyphName].changed()
def closeCallback(self, sender):
font = CurrentFont()
if font is None:
return
for glyph in font:
if not self.key in glyph.lib:
continue
del glyph.lib[self.key]
glyph.changed()
removeObserver(self, "glyphCellDrawBackground")
CustomGlyphCellLabel()