Custom glyph preview ↩
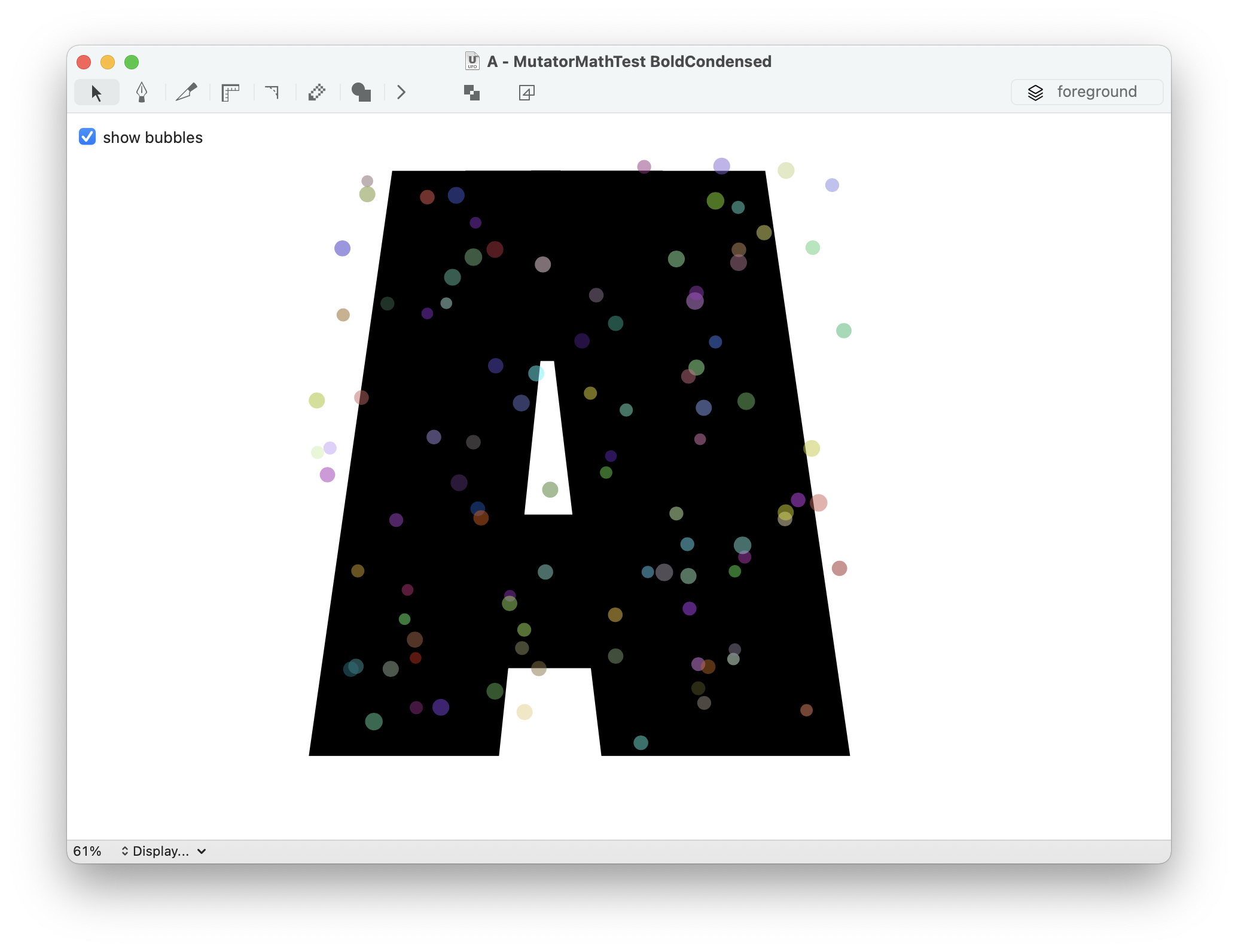
This example shows how to draw something in the canvas when previewing a glyph.
from random import random, randint
from vanilla import CheckBox
from mojo.roboFont import CurrentGlyph
from mojo.subscriber import Subscriber, registerGlyphEditorSubscriber
class BubblesPreview(Subscriber):
debug = True
def build(self):
self.showBubbles = CheckBox((10, 10, -10, 20),
'show bubbles',
value=True,
callback=self.showBubblesCallback)
self.glyphEditor = self.getGlyphEditor()
self.glyphEditor.addGlyphEditorSubview(self.showBubbles)
self.container = self.glyphEditor.extensionContainer(
identifier="com.roboFont.Bubbles.preview",
location="preview",
clear=True
)
self.bubblesLayer = self.container.appendBaseSublayer()
def glyphEditorDidSetGlyph(self, info):
self.updateBubbles()
def showBubblesCallback(self, sender):
self.bubblesLayer.setVisible(sender.get())
if sender.get():
self.updateBubbles()
def updateBubbles(self):
self.bubblesLayer.clearSublayers()
glyph = CurrentGlyph()
# bail out if no glyph
if glyph is None:
print('sorry, no glyph!')
return
# create bubble (given they have a fixed number, they could be "templated")
xmin, ymin, xmax, ymax = glyph.bounds
for ii in range(10):
for jj in range(10):
diameter = randint(16, 24)
self.bubblesLayer.appendOvalSublayer(
# random position
position=(randint(int(xmin), int(xmax)),
randint(int(ymin), int(ymax))),
# random size
size=(diameter, diameter),
# random color, with fixed alpha
fillColor=(random(), random(), random(), 0.5),
)
if __name__ == '__main__':
registerGlyphEditorSubscriber(BubblesPreview)