Glyph Editor subview ↩
This example shows how to draw or add controls directly into the Glyph Editor.
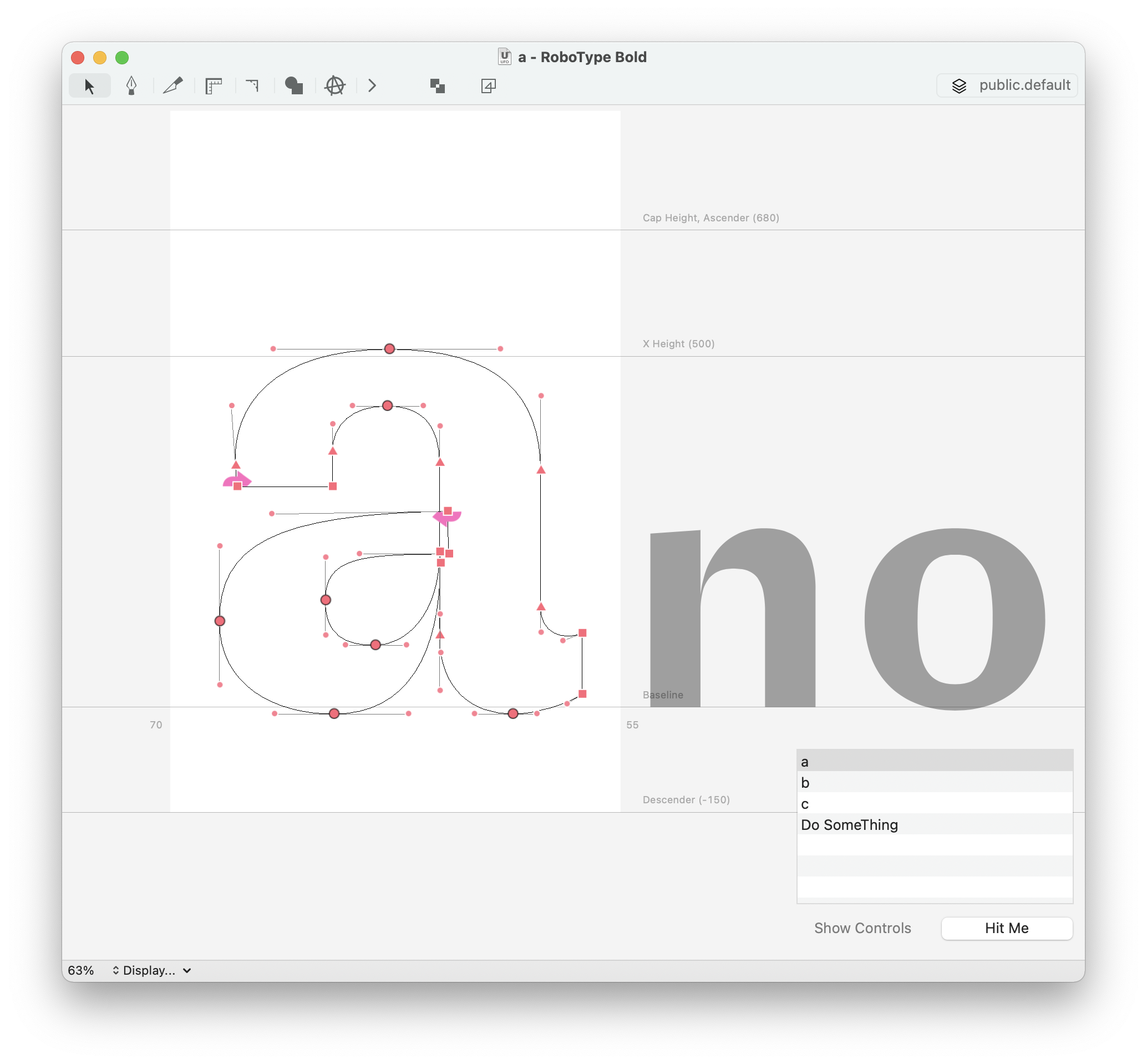
from vanilla import Button, List, Group
from mojo.subscriber import Subscriber, registerGlyphEditorSubscriber
SCALE_FACTOR = 0.5
class OverlayViewController(Subscriber):
debug = True
ctrlGroup = None
def build(self):
glyphEditor = self.getGlyphEditor()
# merz view
self.container = glyphEditor.extensionContainer(
identifier="com.roboFont.OverlayViewController.foreground",
location="foreground",
clear=True)
# controls
self.ctrlGroup = Group((0, -200, -0, -0))
self.ctrlGroup.button1 = Button((-130, -40, 120, 22),
"Hit Me",
callback=self.button1Callback)
self.ctrlGroup.button2 = Button((-260, -40, 120, 22),
"Show Controls",
callback=self.button2Callback)
self.ctrlGroup.button2.getNSButton().setShowsBorderOnlyWhileMouseInside_(True)
self.ctrlGroup.list = List((-260, 10, -10, -50),
["a", "b", "c", "Do SomeThing"])
# add the view to the GlyphEditor
glyphEditor.addGlyphEditorSubview(self.ctrlGroup)
def destroy(self):
self.container.clearSublayers()
def glyphEditorGlyphDidChange(self, info):
glyph = info["glyph"]
if glyph is None:
return
self.updateLettersPreview(glyph)
def glyphEditorDidSetGlyph(self, info):
glyph = info["glyph"]
if glyph is None:
return
self.updateLettersPreview(glyph)
def updateLettersPreview(self, glyph):
self.container.clearSublayers()
referenceGlyphs = [glyph.font[nn] for nn in "no" if nn in glyph.font]
with self.container.sublayerGroup():
self.container.addSublayerScaleTransformation(SCALE_FACTOR)
advancement = glyph.width/SCALE_FACTOR
for eachGlyph in referenceGlyphs:
glyphPath = eachGlyph.getRepresentation("merz.CGPath")
glyphView = self.container.appendPathSublayer(
fillColor=(0, 0, 0, .35),
position=(advancement, 0),
name=f'{eachGlyph.name}_layer'
)
glyphView.setPath(glyphPath)
advancement += eachGlyph.width
# button callbacks
def button1Callback(self, sender):
print('button 1 pressed')
def button2Callback(self, sender):
print('button 2 pressed')
if __name__ == '__main__':
registerGlyphEditorSubscriber(OverlayViewController)